![[object Object]](https://i0.wp.com/getmimo.wpcomstaging.com/wp-content/uploads/2024/06/framework_header.png?fit=1920%2C1080&ssl=1)
What Is a Framework in Programming? Definition and the Ultimate Guide
A framework is a set of pre-written code, guidelines, and tools that simplify software development. Explore our 101 guide to frameworks.
Table of Contents
What is a Framework?
What is a framework in programming?
Why do developers use frameworks?
How do frameworks work?
Key types of frameworks
Front-End Web Development Frameworks
React
Next.js
Vue.js
Angular
Other front-end development frameworks
Back-End Web Development Frameworks
Spring
Django
Node.js
Express.js
Ruby on Rails
Other back-end frameworks
Mobile Development Frameworks
Flutter
React Native
Cordova
Unity
Other mobile development frameworks
Data Science Frameworks
Scikit-learn
TensorFlow
Keras
Pandas
Testing Frameworks
Selenium
JUnit / NUnit
Appium
Enterprise Application Frameworks
Spring Boot (Java)
Microsoft .NET
IBM iSeries (RPG)
Frameworks vs Libraries
How to Choose the Right Framework
Get Started with Frameworks
What is a Framework?
A framework is a set of pre-written code, guidelines, and tools that simplify software development. Explore our 101 beginner’s guide to frameworks.
What is a framework in programming?
A software framework is a collection of pre-written code and rules that simplifies and speeds up software development.
It creates a structure for your code and lets you focus on specific features of your project instead of writing everything from scratch.
You can then expand this structure into something useful and build web apps, websites, native apps, and everything in between.
Each framework is typically associated with specific programming languages (e.g., JavaScript frameworks like React and Angular) and particular types of applications (e.g., web apps).
Why do developers use frameworks?
Software frameworks help you at each stage of the development process—from back-end to front-end.
They’re so popular because they allow for:
- Simplified development: Software frameworks provide you with pre-written components and guidelines, reducing development time and helping you focus on more strategic and creative tasks.
- Improved code quality: They often enforce coding standards, rules, and best practices, leading to more organized and standardized foundational code.
- Reduced errors: Built-in security features and framework error-handling mechanisms help prevent code bugs and increase application stability.
- Collaboration: Each framework has a community with shared knowledge and best practices, making learning and fixing errors easy.
In other words, they help you work faster and make sure your foundational code is error-free.
How do frameworks work?
Software frameworks provide developers with reusable code and tools.
When you use a framework, you follow its guidelines that help you organize your code more efficiently.
For example, they usually include modules for common tasks like database access.
Meaning you won’t have to perform them manually—instead, you can call the relevant framework functions.
There are several key aspects of each software framework:
- Reusable components, libraries, and APIs that developers use to add functionality to their applications.
- Standardized structure that ensures consistency and makes it easier for other developers to understand and work with code. This includes directories for models, views, and controllers in MVC (Model-View-Controller) frameworks, which helps organize your code.
- Built-in debugging and testing tools that help developers find and fix errors.
- Adaptability that allows you to customize frameworks for each specific project.
Frameworks are usually created by experienced developers and organizations to solve common issues and improve coding processes.
Key types of frameworks
Various frameworks address specific needs in software development.
For example, web development frameworks help build web apps, while mobile development frameworks support cross-platform applications.
New frameworks always appear, and there are dozens of options to choose from depending on your project.
Let’s explore each framework type in detail and list their key characteristics.
Front-End Web Development Frameworks
Front-end development frameworks help front-end and web developers build websites and web applications.
According to the report by The Software House, the most popular frameworks in this group include React, Next.js, Vue.js, and Angular.
React
React is a JavaScript library developed by Facebook for building user interfaces.
While it’s a library, it’s so popular that it’s usually included in the list of frameworks.
React is known for its Virtual DOM (Document Object Model) and efficient rendering. The Virtual DOM is a behind-the-scenes copy of the actual webpage structure.
When you add changes to your code, React compares the Virtual DOM to the real DOM. It then identifies the best way only to update the specific elements that were modified.
This helps your web pages load and respond faster.
Best for: Interactive user interfaces, complex web applications, high-traffic websites, single-page apps.
Next.js
Next.js is a framework built on top of React that simplifies building complex and scalable web applications.
It extends React’s capabilities and offers features that enhance SEO through server-side rendering (SSR) and static site generation (SSG).
It also boosts speed and responsiveness via automating code splitting.
Best for: Fast and flexible web applications.
Vue.js
Vue.js is another JavaScript framework popular for its ease of use and flexibility, making it a great choice for beginner developers.
It balances between being a full-fledged framework with powerful features and a lightweight library for simpler projects. So it’s easy to adapt it to your specific needs.
Best for: Interactive web interfaces, single-page applications, and various user interfaces.
Angular
Angular is a JavaScript framework for building web applications. It offers a structured approach that promotes code clarity and scalability for complex applications.
This framework is packed with tools for routing, dependency injection, forms management, and more, which reduces the need for external libraries.
Best for: Enterprise-level applications, large-scale apps, and complex projects.
Other front-end development frameworks
Other popular frameworks you can use include:
- Svelte: A lightweight JavaScript framework that compiles your code into highly optimized, fast, and efficient JavaScript, perfect for building fast and scalable web applications.
- Gatsby: A React-based framework that uses a plugin architecture to build fast and SEO-friendly web applications, focusing on server-side rendering and static site generation.
- Nuxt.js: A Vue.js-based framework that provides powerful features for building web applications, including server-side rendering and static site generation.
- Remix: A JavaScript framework that lets you build server-side rendered web applications using modern JavaScript and HTML.
- Ember.js: A JavaScript framework used for building complex web applications, offering a powerful router, dependency injection, and a large ecosystem of plugins.
- Backbone.js: Another JavaScript framework with a lightweight and flexible structure for building web apps.
- Bootstrap: A CSS framework that offers pre-built UI components and a grid system for building responsive web applications, ideal for rapid prototyping and development.
- Semantic UI: Another CSS framework that provides pre-built UI components and a natural language syntax for building web applications.
Back-end web development frameworks
Back-end frameworks help developers build the server-side functionality of web applications and handle tasks on the web server.
Spring
Spring is a Java framework that provides a feature-rich environment for building enterprise-level applications.
It facilitates dependency injection, offers robust tools for transaction management, and provides extensive support for web and data access.
Django
Django is a high-level Python web framework popular for its robust tools for rapid development (helping you build complex applications faster).
It prioritizes security and scales well for large applications.
Best for: large-scale apps and projects requiring enhanced security and complexity.
Node.js
Node.js is an open-source, cross-platform runtime environment built on Chrome’s V8 JavaScript engine.
It’s not exactly a framework but rather the software that lets you run JavaScript code outside of a web browser (on a server).
In other words, it provides the runtime environment for frameworks built on top of JavaScript, letting them leverage Node.js capabilities to create web applications and APIs.
It unifies JavaScript for front-end and back-end development and features a vast collection of pre-written modules (libraries).
Best for: Real-time applications (e.g., collaborative tools) and flexible applications.
Express.js
Express.js is a flexible Node.js framework that helps you build web applications and APIs quickly. It creates a solid foundation for developers and allows for enhanced customization.
Best for: Web applications and APIs that prioritize flexibility and customization, as well as projects where you want more control over the underlying Node.js functionalities.
Ruby on Rails
Ruby on Rails is another high-level web framework built on the Ruby programming language.
It offers features for rapid development and promotes a structured approach with clear coding conventions.
Best for: Complex web applications, prototyping ideas
Other back-end frameworks:
- Laravel: A PHP-based framework that provides tools for building scalable and secure web applications, focusing on flexibility and ease of use.
- Spring Boot: A Java-based framework that simplifies creating production-ready apps with minimal configuration.
- Flask: A lightweight Python framework offering flexibility for building simple and complex web applications.
- ASP.NET Core: An open-source, high-performance web framework for building web applications on Windows using C#.
- NestJS: A JavaScript framework for building scalable and efficient server-side applications leveraging concepts and design patterns inspired by Angular principles.
- Meteor.js: A full-stack JavaScript framework that simplifies building real-time web applications with automatic data synchronization.
- CakePHP: A rapid development framework for PHP that promotes clean code practices and convention over configuration.
Mobile development frameworks
Mobile development frameworks aid developers in creating native apps for smartphones and tablets. Some of the most popular options include:
Flutter
Flutter is an open-source UI framework from Google that uses the Dart programming language.
It lets you create high-performing native-like apps for mobile, web, and desktop with a single codebase.
Best for: Complex and visually appealing apps with a focus on cross-platform development.
React Native
React Native is a popular framework built on JavaScript and React. It’s easy to use and helps developers build native mobile apps for iOS and Android.
Best for: Cross-platform mobile apps
Cordova
Cordova (PhoneGap) is a framework that uses existing web coding languages (HTML, CSS, JavaScript) to build mobile apps.
It acts as a wrapper for these web technologies, allowing them to access native device functionalities.
Best for: Rapid prototyping, simple mobile apps, or situations where web developers want to leverage those skills to build mobile apps.
Unity
Unity is a game engine used to build 2D and 3D games for mobile, PC, console, and other platforms. It offers powerful tools for creating immersive game experiences.
Best for: High-quality 2D and 3D mobile games.
Other mobile development frameworks:
- Xamarin: An open-source framework for developing native and high-performance Android, iOS, macOS, tvOS, and watchOS apps using .NET and C# programming languages.
- Ionic: A popular open-source framework for designing Android and iOS apps using frontend languages like HTML, CSS, and JavaScript.
- Swiftic: Another mobile app development framework for creating cross-platform apps using Swift and Objective-C.
- NativeScript: A cross-platform framework for building mobile applications using JavaScript and TypeScript, popular for its ease of use and extensive plugin ecosystem.
- SwiftUI: An iOS development framework for creating cross-platform apps using Swift, known for its ease of use and high performance.
Data science Frameworks
Data science frameworks are essential toolkits for data scientists. They automate data cleaning, analysis, and machine learning model-building tasks. Here are some of the top examples:
Scikit-learn
Scikit-learn is a user-friendly Python library offering algorithms for common machine-learning tasks. It’s ideal for beginners and projects that don’t require sophisticated deep learning.
Best for: Getting started with machine learning, prototyping models, and general-purpose machine learning tasks.
TensorFlow
TensorFlow is an open-source library used for complex deep-learning models and large-scale data processing.
Best for: Complex deep learning projects, research in machine learning, and large-scale data processing tasks.
Keras
Keras is an open-source neural network library written in Python that can run on top of other popular lower-level libraries like TensorFlow, Theano, and CNTK.
Best for: Rapid prototyping of deep learning models and projects requiring a user-friendly approach to deep learning.
Pandas
Pandas is an open-source software library written for Python. It offers data structures and operations for manipulating numerical tables and time series, which is particularly useful for handling incomplete and unlabeled data
Best for: Data cleaning, data exploration, data preparation for analysis
Testing Frameworks
Testing frameworks include tools, libraries, and practices that streamline software testing. Some of the most popular frameworks in this category include:
Selenium
Selenium is an open-source browser automation framework that lets you write test cases for web applications across various browsers.
JUnit (Java) / NUnit (C#)
It’s a unit testing framework designed for Java and C# programming languages. They offer features for creating isolated unit tests that focus on individual units of code (functions, classes).
Appium
Appium is an open-source mobile testing framework built on top of Selenium. It allows you to automate testing for native, web, and hybrid mobile apps on various platforms (iOS, Android).
Enterprise application frameworks
Finally, enterprise application frameworks help streamline the development of complex software applications in large organizations.
They focus on serving the specific needs of enterprise-level systems.
Here are some examples:
Spring Boot (Java)
Spring Boot is a popular open-source framework for building modern microservices and enterprise applications in Java.
Microsoft .NET
It’s a development platform from Microsoft that includes frameworks like ASP.NET for building web applications and Windows Forms for desktop applications.
IBM iSeries (RPG)
IBM iSeries is a platform for developing business applications that run on IBM iSeries servers.
Frameworks vs Libraries

Libraries and frameworks are commonly confused with each other, but those are two different types of developer tools.
Both libraries and frameworks are collections of pre-written code that solve common coding and streamline development.
However, they differ in their approach and the level of control they offer developers.
- A framework (e.g., Angular and Django) offers full-scale architecture and a set of conventions for building applications. Frameworks usually help you build your app’s overall structure and workflow, and then you write your code according to the framework’s patterns and guidelines.
- A library (e.g., React and jQuery) is a collection of reusable code that typically focuses on a specific task or functionality. You can import libraries into your codebase and use them as you need within your own code.
So, frameworks are well-suited for larger, more complex applications where it’s important to strictly follow best practices. But they can limit how much control you have over your code.
Libraries, on the other hand, work best for smaller projects and give you more flexibility.
How to choose the right framework
Your choice of the best framework will ultimately depend on the project requirements and the specific context.
For example, are you developing a web app or a native app? Which coding languages do you know? And what’s your level of experience?
Overall, there are a few factors you should consider when selecting a framework:
- Community: A strong and active community around a framework is extremely important, especially if you’re a beginner. Look for frameworks with a large and engaged user base, active development, comprehensive documentation, and lots of third-party libraries and tools.
- Scalability and performance: Analyze the framework’s ability to scale and handle complex workloads as your application grows.
- Maturity and stability: Opt for frameworks with a proven track record of stability and reliability. Mature frameworks usually undergo extensive testing, bug fixing, and optimization. However, sometimes, it’s also a good idea to look into newer frameworks, especially for specific, niche use cases.
- Developer experience: Consider the framework’s learning curve. Frameworks with a gentle learning curve and clear documentation will save you much time and effort.
- Security and maintenance: Choose frameworks that prioritize security best practices, receive regular security updates, and have an established process for addressing vulnerabilities.
Finally, always look for a framework that aligns with your specific project.
Check the programming language it serves, the target platform, and any specific features you might need.
Get started with frameworks
Software frameworks provide developers and data scientists with pre-written code, libraries, tools, and guidelines that simplify development and data analysis.
While libraries and frameworks have some overlap, frameworks provide a more comprehensive structure for building mobile and web applications.
Choosing the right framework depends on various factors, from project specifications to your current development skills.
Looking to master essential frameworks used in front-end and back-end development?
Try Mimo’s full-stack development career path — a flexible online course where you can learn coding at your own pace and build a real-life portfolio.
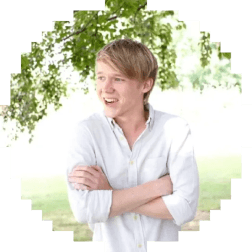