![[object Object]](https://i0.wp.com/getmimo.wpcomstaging.com/wp-content/uploads/2024/06/react_header.png?fit=1920%2C1080&ssl=1)
React vs React Native: Key Differences and Features
Have you ever wondered if React and React Native are the same thing? While these libraries share some similarities, they serve different purposes. In this guide, we dive into the specifics of React vs. React Native.
Have you ever wondered if React and React Native are the same thing? While these libraries share some similarities, they serve different purposes. React.js helps developers create dynamic user interfaces (UIs) for web applications. React Native is used to build mobile apps using JavaScript and React concepts.
In this guide, we’ll dive into the specifics of React vs. React Native and explore:
- What React.js and React Native are
- Their differences and similarities
- Which tool to choose and how to use them both
What Is React?
React (or React.js) is a popular open-source web development JavaScript library that simplifies building user interfaces (UIs) for web applications. Imagine creating a complex user interface (UI) for a website, like a to-do list web app.
Traditionally, front-end developers relied on plain JavaScript to manipulate web pages element by element. They had to write a lot of code to update each item on the list individually.
React.js makes this easier by letting you create reusable building blocks called components, which you can reuse throughout your application.
- Each component controls a specific part of the user interface, like a button or a list item.
- You can define these components with simple instructions, and React.js helps put everything together and keep it up-to-date on the screen.
Here’s a simple example to showcase the difference:
Without React (using plain JavaScript):

With React:

Here’s the explanation:
Imagine displaying a heading. The difference between JavaScript and React would be:
- Without React: We find the element by ID (like a box) and update its content (like putting text inside), which can be clunky for frequent updates.
- With React: We create reusable building blocks called components (like pre-made building blocks). These components take input (like instructions) to display content.
💡 And what if you wanted to change the heading text? React.js uses a concept called virtual DOM (Document Object Model), which helps you automatically update real content on the screen.
When to Use React.js
Use React.js when:
- Your web UI has many changing parts that need to be updated frequently (e.g., live chat, stock stickers).
- You need to keep your code clean and organized for big projects with complex user interfaces (e.g., large e-commerce websites).
- You want to benefit from a wide range of resources and support for web development.
What Is React Native?
React Native is a framework built on top of JavaScript that lets you create native mobile applications for iOS and Android devices. Just like React.js, it’s an open-source tool allowing code reuse in application development.
React.js was created by Facebook in 2013 to address challenges in building and maintaining complex web UI components. React Native emerged a few years later based on React’s concepts. It helps developers build mobile apps using JavaScript and native components.
Key difference: React vs React Native
While React.js focuses on building user interfaces (UIs) for websites, React Native builds UIs specifically for mobile apps. The primary difference lies in how they render the UI:
React.js: Uses web elements (like HTML buttons) suitable for web browsers and focuses on creating web apps.
React Native: Leverages native components built-in to iOS or Android and helps you create mobile apps.
Main similarities of React and React Native
However, React and React Native have many similar features. Both use JavaScript for development. Both emphasize using reusable components to build user interfaces. Both have large and active communities of developers.
Here’s what it looks like in the code:

In this basic example, we create a simple button that says, “Click Me!”
- We import the necessary components (View, Text, Button) from react-native.
- We define a functional component named App.
- Inside App, we return a View container holding our UI elements:
- A Text element displays the message.
- A Button element with the title and an onPress action (logs a message when pressed).
What is React Native for Web (RNW)
While React Native is primarily for building mobile apps, there’s an extension called React Native for Web; It allows you to use some React Native concepts for web development.
RNW provides compatibility between React Native components and APIs with React DOM, the standard library for building web applications with React. This means you can write code using React Native syntax and have it rendered on a web browser.
React Native vs React.js: 7 Primary Differences
As you can see, React.js and React Native share similar core concepts and use JavaScript. However, they cater to different development needs.
React.js | React Native | |
Target Platform | Building web applications | Building native mobile applications (However, you can also use React Native for Web) |
Installation | Using <script> tags or bundlers | IDEs like Android Studio and Xcode |
Development Environment | Code editors and debuggers | IDEs like Android Studio and Xcode or physical devices for testing |
Core Technology | Virtual DOM | Virtual DOM-like mechanism and native APIs |
Elements | Web elements: HTML, CSS, Javascript | Native components (for iOS and Android) |
Syntax | JSX | JSX with its own set of elements |
Learning Curve | Easy if you already know HTML, CSS, and JavaScript | Requires additional knowledge of mobile-specific concepts |
Now, we’ll dive deeper into the key features that set them apart and explain each of them in detail.
1. Target Platform:

First, React.js and React Native run on different platforms:
React.js: Focuses on building user interfaces (UIs) for web applications (software programs you can access and interact with through a web browser).
The web browser acts as a middleman, parsing the code (HTML), applying styles (CSS), and executing JavaScript to render the final web page you see.
React.js further enhances the user experience by dynamically manipulating the web page’s content (the DOM) based on user interactions or data changes.
React Native: Targets native mobile applications for iOS and Android devices.
It uses native UI components that are pre-built into each platform. Unlike web apps, React Native directly interacts with the device’s hardware and operating system.
2. Installation:

React.js: Getting started with React.js for web development is relatively straightforward.
- The traditional method: You can integrate React into an HTML page by manually adding the React and React DOM libraries using <script> tags within the HTML. This method works best for small projects or learning purposes.
Here’s an example:

- The modern method: For larger React.js projects, consider using a framework like Next.js, which is officially recommended by React.
Next.js simplifies setup and provides features like server-side rendering and static site generation, enhancing performance and SEO. Alternatively, you can use a bundler—a tool that combines your JavaScript code, CSS, and other assets into optimized files for your web application. Popular bundlers include Webpack and Rollup.
React Native: Building mobile apps with React Native requires a slightly more complex setup process. Since you’re targeting native mobile platforms (Android and iOS), you’ll need additional tools and configurations specific to each mobile development environment. Popular options include Android Studio for Android development and Xcode for iOS development. These tools provide access to native APIs and native UI components, helping you create mobile apps that feel natural on each platform.
3. Development Environment:

React.js: Lets you leverage existing web development tools like code editors and debuggers. React.js applications run directly in a web browser during the development process. You can also use Node.js servers for more complex web applications that require server-side logic.
React Native: Requires a more specialized setup for mobile app development. While you’ll still use JavaScript code, you’ll need additional tools and configurations to build and test React Native apps. For example, you can use integrated development environments (IDEs) or physical devices for testing. Here again, you can use Android Studio and Xcode.
4. Core Technology:

React.js: Uses the Virtual DOM.
The DOM (Document Object Model) is a tree-like structure that represents all the elements on your webpage, like headings, paragraphs, buttons, and images. The Virtual DOM is a temporary, lightweight copy of the real DOM. When you make changes to your web app, React.js first updates the Virtual DOM. Then, it compares the updated Virtual DOM to the real DOM and only applies the minimal changes necessary to the real DOM.
React Native: Uses a virtual DOM-like mechanism and native APIs to render mobile components. It maintains a virtual DOM-like structure to manage its UI components. Instead of targeting a web browser’s DOM, it syncs and communicates with native UI components provided by the Android or iOS platform.
5. Elements:

As you can see, React and React Native also use different components:
React.js: Uses web elements, which are essentially HTML elements enhanced with CSS styling and JavaScript interactivity.
React Native: Focuses on native app components built-in to the target mobile platform (iOS or Android).
6. Syntax:

React.js: Syntax is a set of rules that define how a programming language is structured. React.js code is written in JavaScript and uses JSX, a syntax extension that lets you write HTML-like structures within your code. Here’s an example of a simple component written in JSX:

React Native: React Native uses JSX just like React, but instead of using HTML-like tags, it leverages its own set of elements that map to native UI elements. For example, instead of using <div> and <p>, React Native uses <View> and <Text> to create native mobile UI components.
7. Learning Curve:

React.js Getting started with React.js for web development has a gentler learning curve. It’ll be a natural extension if you’re already familiar with front-end languages like HTML, CSS, and JavaScript.
React Native: Building mobile apps with React Native requires a bit more knowledge compared to React.js. While a solid understanding of JavaScript is essential for both, React Native involves concepts specific to mobile development.
For example, using pre-built mobile components or adhering to platform guidelines. In other words, the complexity of what you need to learn depends on the complexity of the native applications you’re building.
FAQs about React Native and React.js
Now, let’s answer the most popular questions about React and React Native.
Is React Native the Same as JavaScript?
No, React Native is not the same as JavaScript. React Native uses JavaScript as its primary programming language. But it’s a separate framework for building native mobile applications.
React Native | JavaScript |
React Native is a framework for mobile application development using React and JavaScript. | JavaScript is a programming language used for web development. |
React Native uses native components and APIs to create mobile apps that look and feel like native apps. | JavaScript helps you build interactive web pages and applications that run in web browsers. |
React Native uses native APIs to render components in mobile apps. | JavaScript adds nteractivity and dynamic behavior to web pages. |
Which is Better, React Native or React.Js?
The choice between React Native and React.js depends on your specific project requirements and goals. For example:
- Enterprises with extensive codebases may choose to continue investing in React.js for web development while using React Native for mobile applications.
- React Native is a better choice for developing cross-platform native mobile applications.
- React.js is the preferred option for building complex single-page web applications, portals, and web UIs that require high interactivity and frequent updates.
- React Native has a slight edge for performance-critical mobile apps due to its closer integration with native platforms.
- React Native might also be more appealing to startups and small to medium-sized businesses looking to establish a presence on both mobile and web platforms.
Should I learn ReactJs or React Native first?
Similarly to the previous question, this choice will depend on your priorities.
React.js is a better starting point if:
- You want to build complex web UIs or web applications with large existing codebases.
- You are already familiar with JavaScript and want to focus on learning React’s specific concepts and tools.
- You want to start with a more established technology in web development.
React Native is a better starting point if:
- You want to build cross-platform native mobile applications for iOS and Android.
- You are interested in mobile-first development and want to use React’s capabilities to build mobile apps.
Note that React Native is gaining growing popularity in 2024.
Should You Use React Native for Web or React.js?
You will often use React.js to build web applications and React Native to create mobile applications for Android and/or iOS devices. However, you can also use React Native Web (RNW) for projects that require sharing significant code with your React Native app.
Is React Native Outdated?
React Native is a framework that’s constantly evolving and developing. While occasional outdated components may exist, the community actively maintains and updates the platform. It embraces modern JavaScript features and integrates well with various popular tools and the latest React versions.
Should You Learn React?
Learning React is a must for developers looking to build user interfaces and web applications. It’s a popular library used by major companies Meta, Netflix, Uber, etc. According to the report by The Software House, React is the top framework used by front-end developers.
Learning React will also help you understand key concepts like JSX, components, state, props, and hooks—essential for creating effective web apps.
Learn more about becoming a front-end developer in our detailed guide.
How to Learn React?
There are several ways to learn React. First, you can use various online materials and benefit from the active developer community. However, it’s best to combine them with consistent, guided learning.
Use online front-end development courses like the Mimo platform offers. Our interactive online platform lets you explore the topic at your own pace as well as practice coding immediately.
Is it Worth Learning React Native?
Learning React Native can also be extremely useful for developers as it lets you create cross-platform mobile applications using a single codebase.
Learn React Native if:
- You want to build mobile apps (which are in high demand!).
- You already know JavaScript
- You value fast development (single codebase for Android & iOS).
React Native is gaining a lot of traction and interest in 2024. Based on Google Trends data, conversations about this topic have been on the rise over the past few years.
Overall, there’s a lot of demand for React developers on the market. For example, as of May 2024, there were over 4,5K relevant job offers on Indeed.com in the US alone.
Does React Native Have a Future?
React Native seems to have a bright and promising future. The framework continues to improve its compatibility with modern tech trends like AI, machine learning, and cloud computing. Combining it with React.js and other front-end development skills creates a strong base for growing your career in programming.
Should You Learn Django or React?
Django is a free and open-source Python web framework for building web applications. There are several important differences to keep in mind:
- Django is ideal for back-end development and projects requiring complex database operations and scalability.
- React is great for front-end development and creating interactive web applications.
Developers often use Django for back-end development and React for front-end development.
Should You Learn Python or React Native?
React and Python can also complement each other depending on your goals.
- Learn Python for general-purpose programming and back-end development.
- Learn React Native to build mobile apps with a single codebase for Android and iOS (using JavaScript).
The Bottom Line
As you can see, React and React Native share many similarities. However, they target different platforms and use cases:
- Choose React.js for interactive web applications with frequent updates (e.g., e-commerce websites, live chat features).
- Choose React Native to build native mobile apps for iOS and Android using a single codebase (if you know JavaScript).
React and React Native offer great career prospects and are worth learning—depending on your goals and interests.
Ready to give it a try?
Start learning React today with Mimo’s Front-End development career path. You’ll get access to a flexible, interactive learning experience to help you master React and build a career in tech.
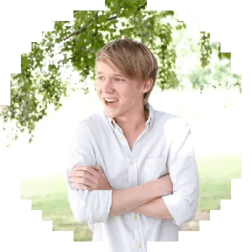